Factorial Program In Java Using Thread
Posted By admin On 24.01.20Hey again guys, I have a new programming assignment asking me to make a program that will, based on user input, either find the factorial of a number or find BASE raised to the power of the number read in. And for this particular programming assignment, BASE is a named constant equal to 2, which could be changed later on if desired. It will continue to run until the user enters 'quit' to quit the program. The commands 'factorial', 'power', and 'quit' must be named constants in the program and this assignment suggests the constant variables be named COMMAND1, COMMAND2, and COMMAND3. We aren't allowed to use the words FACTORIAL, POWER, or QUIT as the constant variable names.
It says I will have to enter at least one loop to continue until 'quit' is entered. If a user enters the text 'factorial' it has to call the method factorial which has the header: public static int factorial(int iVal, boolean DEBUG) In the factorial method, it needs to verify the number is greater than 0, and if the condition is met it finds and returns the factorial of the number read in. If the condition is not met, it has to print an error message and terminate the program. It gives an example that reads: If 4 is inputted than 4! = 4. 3. 2.
1 with a result of 24 It also says I might want to think about a loop to complete this. If a user enters 'power' I need to call the method power which has the following header: public static int power(int iVal, int BASE, boolean DEBUG) In this method, I need to verify that the integer is also greater than 0; if the condition is met then it needs to be able to compute and return BASE raised to the value iVal. If the condition is not met, it needs to print an error message and terminate the program. It gives an example here: If 3 is read in and BASE is equal to 2, then 2 ^ 3 = 2.
2. 2 = 8 It says a loop is required to calculate this result The program instructs that we are NOT allowed to use Math.pow anywhere in this program and that it is not necessary. If we do we lose credit.
If the user inputs anything other than 'factorial', 'power' or 'quit', it displays an error message and terminates the program. It recommends against using recursion in this program if I am a noob, which I am. It also states 'in mathematis (not sure if it was supposed to be 'mathematics' or spelled how it is) like 0! (zero factorial) is defined, a positive BASE raised to a zero power is defined, and a negative power is defined. If we allow a type double result, but for simplicity of the programming assignment we are not handling those values.' Here is the skeleton of the program I have so far. (for the updated code, look to the latest posts).
Well, what is a factorial and an exponent? A factorial is a number times every number smaller than it until 1.
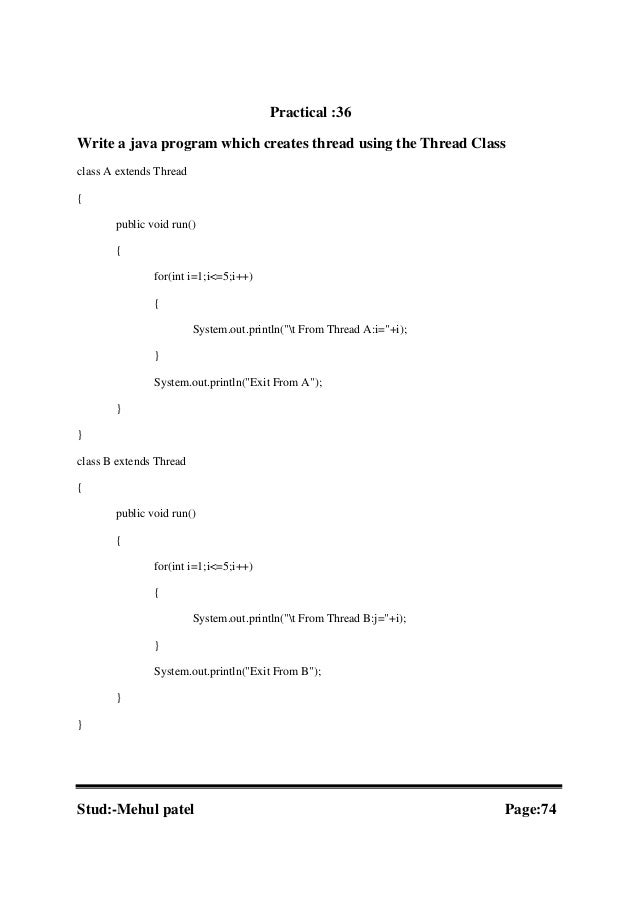
If you have a starting number, and you want to go through all numbers until 1, what construct in Java should you use? (Hint, it is the only loop you use if you know the number of times it should happen) Powers are basically the same, but take a number and multiply it by itself a certain number of times. Use the same loop as above. You will use a different type of loop to keep going until the user tells you to quit. Since there are only two loops, this should be plenty to get you started DO NOT use recursion if you are not extremely comfortable with it. So uh, don't remove comments.
They are essentially mandatory everywhere. Just tab them over, or consolidate them into a header for methods/loops/files/classes and anything else that needs explaining or isn't obvious. As for your implementation, don't forget that factorials don't work on negative numbers, and that 0!
Also, don't forget that powers have. Odd interactions below/at 0. N^0 is always 1, and n^(-m) is always 1/(n^m). If you need more help, I'll keep an eye on this thread whilst I work on my compiler for the next few hours. A couple more things.
Your methods probably shouldn't be 'int' methods. For factorials and powers, they both can return floating point values. If you only take in integers, factorials will always be integral, but can quickly be large enough to need to be 'long' as a result. The java 'int' type overflows at 2,147,483,647 (there's no unsigned int in java as far as I know.) 13! Is 6,227,020,800 and is the first factorial that will easily overflow your int into negative numbers. A 'long' won't overflow until 9,223,372,036,854,775,807, which is any factorial under 21!
I'd use a 'long' for factorial, and throw an error if they enter anything above 20. As for powers, even taking in integers can cause you to see some really interesting things. I'd personally have 2 powers methods, one for negative powers that returns 'double' (float isn't tiny.
But you don't know how crazy the input is going to be), and a 'long' one. If the power is negative, use the 'double' one and return a 'double' result, and if the power is positive, use the 'long' one.
Java Factorial Example
Either that or cast the 'long' one to a 'double' and use that for the math. Something like. Hey Annoying, I am looking at what I have right now and I'm getting errors in the code still just trying to get the backbone of it working. So I'm sorry it is taking forever. Also, I don't think we've learned about overflow errors yet so I don't know how to write that. We've only been working with int and not other types of methods, so I'm not sure it would be acceptable or how I would do anything other than int.
Like I said I'm a complete noob to Java, and I am just now starting to learn about it, so I have a long way to go. Right now I need to look at one thing at a time. So the main thing I am focused on right now is trying to figure out what variables and constants I need for this program to work and why. Then after I get that done, I need to figure out how to get my program to perform a loop as long as 'quit' is not entered, and inside that loop, to only accept the words 'factorial' and 'power'. If anything else is entered it should supply an error message and terminate. As soon as I get these done I will look at the next things to work on.
Java Factorial Math
Thanks again for everyone's help and contributions, I'm looking forward to your help on how to do these things!